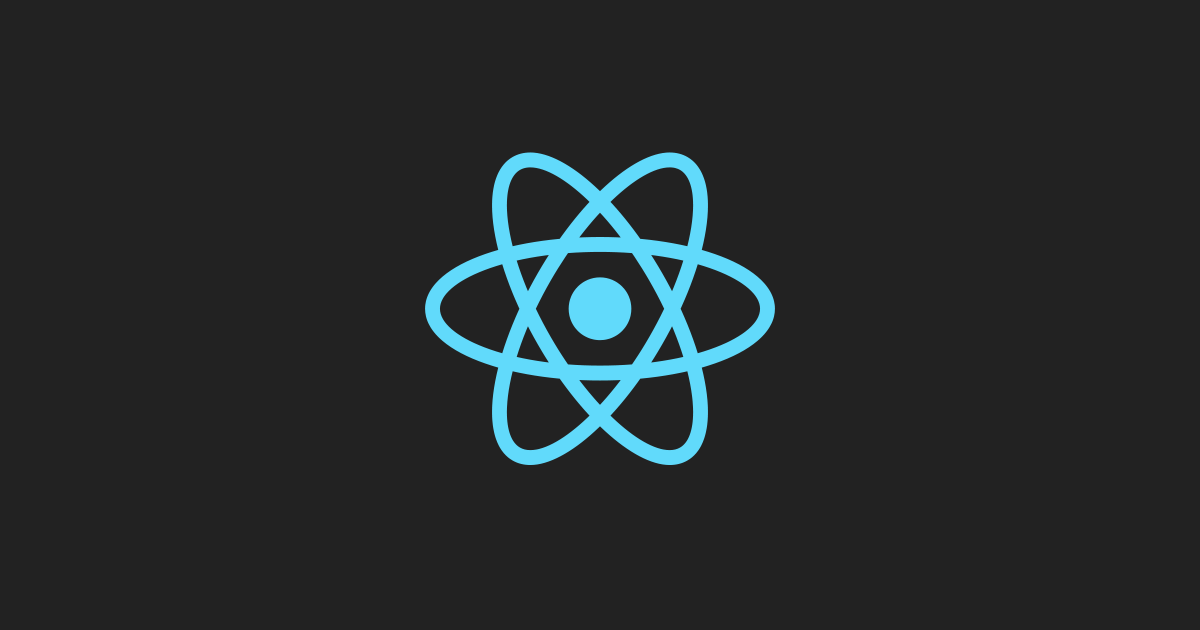
Understanding Component Composition in React
How to use component composition in React to build reusable components.
How to use component composition in React to build reusable components.
How to build authentication in web services using JSON Web Tokens (JWTs) using Express in the backend and React in the frontend.
Axios interceptors provide a powerful tool that can be used to implement middleware-style functionality in your web applications. In this article, we'll explore how Axios interceptors work and how they can be used to modify HTTP requests and responses.
Firebase Authentication provides a secure and easy-to-use way to authenticate users and manage user sessions for your API. In this article, we'll cover the basics of building an API with Firebase Authentication.
When we talk about HTTP requests, most people think about the well-known methods such as GET, POST, PUT, DELETE, etc. However, there’s another method that is also essential in some cases: the OPTIONS method. In this article, we’ll explore what an OPTIONS request is, how it works, how it has evolved throughout the years, and its usage for Cross-Origin Resource Sharing (CORS).
Node.js is a popular server-side runtime environment that allows developers to run JavaScript code outside of a web browser. One of the challenges of working with Node.js is understanding the module systems and how they work. In this post, we’ll explore the differences between CommonJS (CJS) and ECMAScript Modules (ESM), and show you how to start an npm project using the ESM syntax and build an npm module that can be used by both module systems.
As a developer, it's easy to accumulate a large number of GitHub repositories. For me, it can be overwhelming to keep track of them all and remember what each one does. So, I decided to make a list of my most important repositories by categories, along with a brief description of what they are.
Learn the differences between 'reload' and 'no-cache' cache modes in the Fetch API and how they affect web performance. By understanding these cache modes, you can make informed decisions when designing and optimizing your applications to deliver an efficient and responsive user experience.
Upgrade your website’s functionality and design with our “Building Custom Blocks for WordPress” series. Our step-by-step guide is beginner and expert-friendly, ensuring that everyone can create custom blocks that enhance their website’s capabilities. Get ready to take your WordPress website to the next level!
Managing multiple WordPress sites locally can be a challenge, but Local by Flywheel makes it easy. In this post, we'll explore the benefits of this powerful desktop application for WordPress developers.
In this tutorial, we will delve into the intricacies of animating a humanoid model in Unity.
Gutenberg is the latest editor for Wordpress. It uses “blocks” to edit the content of its pages and posts. These blocks can be any different kind of stuff: Text, buttons, heading, carousels, dropdowns, etc…
Let’s take a React component, that simply displays a number. This can be written both as a class component or as a function component.
I just installed the latest Ubuntu release (right now 19.10). These are the first things I do, to set up my system:
I have seen other people writing articles with about the same topic, so I thought I would share as well my set of tools, to compare and to let know others what it is that they could be using.
When you make a new Rails 5 project and take a look at the default routes that come out-of-the-box, you will see something like this:
It is not seldom that a website needs different kinds of data. Wordpress comes out of the box with “post” and “page”, but we might need more.
Lately I started tinkering around with framework for static site generation sites, and I saw something new that I did not know. The so called YAML Front Matter (or just Front Matter).
Programmers usually have a problem when they have to use more than one word to describe something, but they are not allowed to use spaces or punctuation signs. An example of this would be to name files. If someone wants, for instance, to name a picture where 2 people are talking, he would like to write John and Mike talking.jpg
, but since a filename cannot contain spaces, he may write something like john-and-mike-talking.jpg
Today I Learned that there is a thing called “scientific notation literals” in Javascript. Let’s see some examples.
If you have ever open the developer tools and used the console, most likely you have used many times the following snippet to debug your JS code.
I have started a new project in an area that is out of my comfort zone. I am developing my first video-game for the PS4, codename NARG. Today I am going to talk about Scriptable Objects.
All developers need to loop through arrays or lists sooner or later, to perform operations on its items. For instance, let us say that we want to loop through an array of *enemies* to check in any of them are alive.
Changing themes in Sublime Text can be a difficult task. Not because it is extremely critical for your productivity, but we all want our editor to have a proper color, that does not stop our concentration. It is like waking up in the morning and spending 30 minutes in front of the mirror, trying to decide what trousers to wear, so that our ass do not look too fat.
The functions bind and live are being deprecated in jQuery 1.7+ in favor of on, but it’s not very clear how their differences reflect on it. As you might know, bind
attaches event handlers on DOM elements existing at the time of running the instructions, while live
also attaches these handlers to DOM elements added in the future. So how does it work with on
?